Setting different config files in Node.js
External config files
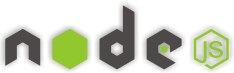
When it comes to configuration options it is always desirable to avoid hardcoding config values in our code. Even more, if we are using different config values in development and production environments it is necessary an easy way to switch from one to another. This also becomes useful when your code is public but you want to hide some config files. In Node.js there is more than one way to do this.
Using config module
Easy to install, easy to configure, easy to use. The module you need is named config and you can have it just doing npm install config
or adding config in the dependencies section of your packages.json file:
{ "name": "world-domination-project", "version": "0.0.1", "private": true, "scripts": { "start": "node app" }, "dependencies": { "express": "~4.2.0", "config": "~0.4.36", "mongoose": "*", "debug": "~0.7.4" } }
The project is hosted on github, and just taking a look to the README you can set everything up.
Creating config files
First of all, in your project root you must create a folder called config with a mkdir config
o whatever. If you love troubles and you don't like to be said what and where do you have to create folders, you'll have to set the $NODE_CONFIG_DIR
variable.
If you want more details just read more about configuration files options. You can pick between many different flavors of config syntax: json, yaml, cofeescript, js,... What I am showing here is quite simple: a default config and a production configuration in json format.
config/default.json
default.json is the first file that config module tries to get. So this is fine for development, and this is how it looks like:
/** * default config file */ { "www" : { "port" : 3000 }, "db": { "host": "localhost", "port": "27017", "dbname": "blog", "username" : "", "password" : "" }, "debug": { "level": 0 } }
config/production.json
Here comes the production config, with the same keys but with different values. As you may expect, ports, hosts, passwords, are different.
{ "www" : { "port" : 80 }, "db": { "host": "imsokweliuse.mongolabs.net", "port": "27017", "dbname": "blog", "username" : "snowden", "password" : "changeme" }, "debug": { "level": 1 } }
Applying config file
Very easily, just require config module and you are ready to go. The json file is loaded and everything is handled in a variable.
If you want to apply the production.json file, you have to set NODE_ENV environment variable to production, this way: export $NODE_ENV=production
. That is something that maybe in your production server is already set. As for the code, behold the config module working:
/** * app.js - pello altadill * @greetz to any */ ... var express = require('express'); var mongoose = require('mongoose'); var mongooseTypes = require("mongoose-types"); var config = require('config'); mongooseTypes.loadTypes(mongoose); console.log("Show conf:" + config.www.port); mongoose.connect('mongodb://'+config.db.username+':'+config.db.password+'@'+config.db.host+':'+config.db.port+'/'+config.db.dbname); var app = express(); ...
Yeah I know, maybe instead of splitting all the database config we should just set one url containing everything. Do it your way.