Posts
In this series of posts I'm trying to show, in the simplest way possible, how to develop a web application in Node.js adding all the features that in other environments we take for granted: database access, form validation, i18n, and of course session management. Express is the framework of choice for this purpose, the problem is that maybe many information you find out there may be deprecated or useless since express 4 was released. Here I'm trying to use the latest (express4) and the coolest, but in any case in the months to come this information may be crappy. As some dear friend of mine says talk is cheap, show me the code, so let's get down to the nitty-gritty details.
In any application, no matter the languages or the environment you are using, it is always necessary to ensure that all data is validated before we try any operation. It's tedious, boring and maybe you prefer to focus on more interesting things but you may fear not only hackers, but also the users who from time to time become true hackers without been really aware of it: just applying unexpected input. For instance a form field could ask for the zip code and a regular user could answer (sincerely) 'I don't know'. And he is being honest but at the same time he is creating an exception and blowing out your super-duper node server.

In addition to that and in order to be nice with a wider range of users you have to offer internationalization support. Once again, a boring but a mandatory task to take into account when you are dealing with the views. In this post I'll show one approach to solve these issues node.js trying to keep the code simple. Let's start with i18n.

This post has two purposes: to continue the previous one showing ways to improve the organization of routes and secondly to see how to add your own middleware to an express application.
The previous version have a problem when it comes to the routes. We require all of them with a single require command but we were applying them one by one.
var routes = require('./routes'); ... // We set routes app.use('/', routes.home); app.use('/guestbook', routes.guestbook);
Use a contructor in routes/index.js
There Is More Than One Way To Do It claims the Programming Perl book on the cover. Something that, undoubtedly, fits Node.js. There is more than one style in Node-foo, and I guess that you have to choose the way that better fits your needs. My need here was to minimize the code to initialize the routes in the main file of the Node.js express application. We change the routes/index.js to have a constructor with a parameter: the express app itself
This is routes/index.js
/** * routes/index.js * All routes are here to import all of them just with one command * in the main app.js * https://github.com/pello-io/simple-express-mongoose * Pello Altadill - http://pello.info */ var home = require('./home'); var guestbook = require('./guestbook');
External config files
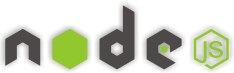
When it comes to configuration options it is always desirable to avoid hardcoding config values in our code. Even more, if we are using different config values in development and production environments it is necessary an easy way to switch from one to another. This also becomes useful when your code is public but you want to hide some config files. In Node.js there is more than one way to do this.

MongoDB is extremely easy to install and run. If you are not able to make it work maybe you could feel more confortable using Access or even excel. Well, sorry for that. This short article is but a quick and dirty guide to enable basic authentication in mongodb, and how to create one database with its own user (not a superadmin) with just read/write permissions.
Python in 21 minutes (or so)
For a developer is not enough to know one programming language, it is often said that is good to know many of them. After all the language is but a mere tool to build programs. Python has been around for many years and one of my pending task was to take a look at it. Some friends (@Eugenia4v,@Claw_Shadow,@D00m3dr4v3n) are always telling me about the benefits of Python not to mention plenty of papers where Python is even recommended as the language of choice to get into programming. I guess that when Google itself has been using Python from the beginning, and when this language is a requirement for many job offers there is something about it. Despite the title of this post, I spent more than 21 minutes coding around and trying the basics so don't expect a deep dive into Python. This is just a quick guide
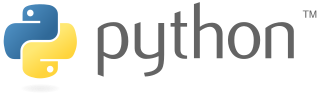
Python is: interpreted, weakly typed, (could be compilated), case sensitive, supports oop, functional, imperative programming,... apart from the classic definition, if you come from c style languages at first glance you'll notice the lack of semicolons. But the real change in Python is the use of indentation to create code blocks. So instead using brackets for if-else-loops-functions you have to use indents or tabs properly. This at least, somehow leads to mandatory code convention (they exists too), here there is no room for discussion about the most clear way to write an if-else structure; there is only "one style" so follow it or your code will not run at all. But to sum up, and above all, python was designed to be easy to read
Lynx Theme for webmin
A night out with JasmineUnit testing is much more than a tool for ensuring that a program behaves as expected. Nowadays it's the key for new development techniques such as TDD and BDD in which you begin writing tests before the required code. It's all about requirements and behaviour; developers must focus on understanding the requirements instead of thinking about the way they implement the code. In the event that you've forgotten the fundamental benefits of unit testing here you have a quick reminder:
![]() This a classic pong-like game that takes some ideas from Arkanoid. Basically two paddles try to hit the ball until one of them fails but, from time to time the rules can change if special bricks are touched.
![]() For one or two players, much more fun if you play it against someone else, the game is controlled with your fingers. You can set your preferred ball speed using the preferences screen. There is another Arkapong out there, not released yet but It looks very interesting. It's a similar concept but this one is more based on Arkanoid subscribe via RSS |